I2C Communication Protocol
I2C terminology
The configuration of I2C on AVR Atmega16
TWBR: TWI Bit Rate Register

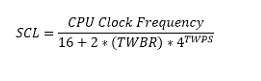
TWSR: TWI Status Register

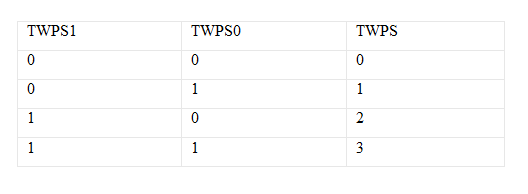
TWCR: TWI Control Register

I2C Operation
1.Taking control of the TWI bus by sending a start signal to it when it is free
2. Start TWI Data Transmission
3. Receive TWI Data
4. Stop transmission and Release the TWI bus
EEPROM Write Data

Applications
/* Name : main.c * Purpose : Source code for EEPROM interface with ATMEGA16. * Author : Gemicates * Date : 2017-09-12 * Website : www.gemicates.org * Revision : None */ #ifndef F_CPU #define F_CPU 8000000UL // 8 MHz clock speed #endif #include<avr/io.h> #include<util/delay.h> void init_i2c() { TWSR = 0X00; TWBR = 0X47; TWCR = (1<<TWEN); } unsigned char read_i2c() { TWCR = (1<<TWINT)|(1<<TWEN); while(!(TWCR & (1<<TWINT))); return TWDR; } void write_i2c(unsigned char ch) { TWDR = ch; TWCR = (1<<TWINT)|(1<<TWEN)|(1<<TWEA); while(!(TWCR&(1<<TWINT))); } void start() { TWCR = (1<<TWINT)|(1<<TWSTA)|(1<<TWEN); while((TWCR &(1<<TWINT))==0); } void stop() { TWCR = (1<<TWINT)|(1<<TWEN)|(1<<TWSTO); _delay_ms(1); } void eeprom_write(char dev_addr,char dev_loc,char dev_data) { start(); write_i2c(dev_addr); write_i2c(dev_loc); write_i2c(dev_data); stop(); _delay_ms(10); } unsigned char eeprom_read(char dev_addr,char dev_loc) { char ch; start(); write_i2c(dev_addr); write_i2c(dev_loc); start(); write_i2c(dev_addr|0x01); ch = read_i2c(); stop(); return ch; } int main() { char ch,i; DDRC =0XFF; init_i2c(); for(i = 0;i<26;i++) eeprom_write(0xa0,i,'A'+i); for(i = 0;i<26;i++) { ch = eeprom_read( 0xa0 , i); eeprom_write(0xa0,i+40,ch); } }